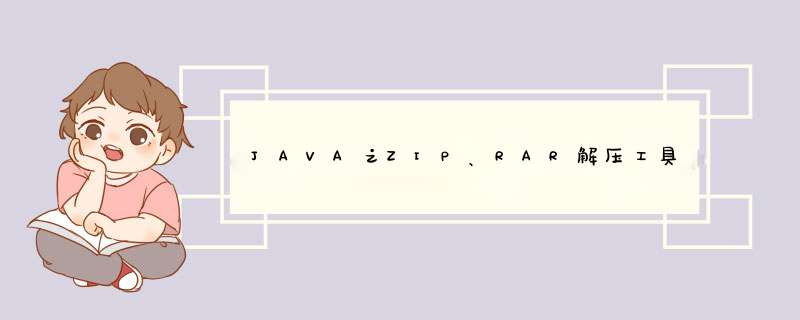
废话不多说直接上代码
1.相关依赖pom
net.sf.sevenzipjbinding
sevenzipjbinding
16.02-2.01
net.sf.sevenzipjbinding
sevenzipjbinding-all-platforms
16.02-2.01
2.解压压缩文件工具类
import net.sf.sevenzipjbinding.IInArchive;
import net.sf.sevenzipjbinding.SevenZip;
import net.sf.sevenzipjbinding.impl.RandomAccessFileInStream;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.*;
import java.nio.charset.Charset;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import java.util.zip.ZipOutputStream;
public class UnZipAndRarUtil {
private static Logger logger = LoggerFactory.getLogger(UnZipAndRarUtil.class);
private static final int BUFFER_SIZE = 2 * 1024;
/**
* 压缩成ZIP 方法1
*
* @param srcDir 压缩文件夹路径
* @param out 压缩文件输出流
* @param KeepDirStructure 是否保留原来的目录结构,true:保留目录结构;
* false:所有文件跑到压缩包根目录下(注意:不保留目录结构可能会出现同名文件,会压缩失败)
* @throws RuntimeException 压缩失败会抛出运行时异常
*/
public static void toZip(String srcDir, OutputStream out, boolean KeepDirStructure) {
long start = System.currentTimeMillis();
ZipOutputStream zos = null;
try {
zos = new ZipOutputStream(out);
File sourceFile = new File(srcDir);
compress(sourceFile, zos, sourceFile.getName(), KeepDirStructure);
long end = System.currentTimeMillis();
logger.debug("压缩完成,耗时:" + (end - start) + " ms");
} catch (Exception e) {
throw new RuntimeException("zip error from ZipUtils", e);
} finally {
if (zos != null) {
try {
zos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
/**
* 压缩成ZIP 方法2
*
* @param srcFiles 需要压缩的文件列表
* @param out 压缩文件输出流
* @throws RuntimeException 压缩失败会抛出运行时异常
*/
public static void toZip(List srcFiles, OutputStream out) {
long start = System.currentTimeMillis();
HashMap
3.创建提取文件实现类(IInArchive类属于SevenZip.Archive包)
import net.sf.sevenzipjbinding.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.*;
public class ExtractCallback implements IArchiveExtractCallback {
private static Logger log = LoggerFactory.getLogger(ExtractCallback.class);
private int index;
private IInArchive inArchive;
private String ourDir;
public ExtractCallback(IInArchive inArchive, String ourDir) {
this.inArchive = inArchive;
this.ourDir = ourDir;
}
@Override
public void setCompleted(long arg0) throws SevenZipException {
}
@Override
public void setTotal(long arg0) throws SevenZipException {
}
@Override
public ISequentialOutStream getStream(int index, ExtractAskMode extractAskMode) throws SevenZipException {
this.index = index;
String path = (String) inArchive.getProperty(index, PropID.PATH);
final boolean isFolder = (boolean) inArchive.getProperty(index, PropID.IS_FOLDER);
String finalPath = path;
File newfile = null;
try {
String fileEnd = "";
//对文件名,文件夹特殊处理
if (finalPath.contains(File.separator)) {
fileEnd = finalPath.substring(finalPath.lastIndexOf(File.separator) + 1);
} else {
fileEnd = finalPath;
}
finalPath = finalPath.substring(0, finalPath.lastIndexOf(File.separator) + 1) + fileEnd;
//目录层级
finalPath = finalPath.replaceAll("\\\\", "/");
String suffixName = "";
int suffixIndex = fileEnd.lastIndexOf(".");
if (suffixIndex != -1) {
suffixName = fileEnd.substring(suffixIndex + 1);
}
newfile = createFile(isFolder, ourDir + finalPath);
final boolean directory = (boolean) inArchive.getProperty(index, PropID.IS_FOLDER);
} catch (Exception e) {
log.error("rar解压失败{}", e.getMessage());
}
File finalFile = newfile;
return data -> {
save2File(finalFile, data);
return data.length;
};
}
@Override
public void prepareOperation(ExtractAskMode arg0) throws SevenZipException {
}
@Override
public void setOperationResult(ExtractOperationResult extractOperationResult) throws SevenZipException {
}
public static File createFile(boolean isFolder, String path) {
//前置是因为空文件时不会创建文件和文件夹
File file = new File(path);
try {
if (!isFolder) {
File parent = file.getParentFile();
if ((!parent.exists())) {
parent.mkdirs();
}
if (!file.exists()) {
file.createNewFile();
}
} else {
if ((!file.exists())) {
file.mkdirs();
}
}
} catch (Exception e) {
log.error("rar创建文件或文件夹失败 {}", e.getMessage());
}
return file;
}
public static boolean save2File(File file, byte[] msg) {
OutputStream fos = null;
try {
fos = new FileOutputStream(file, true);
fos.write(msg);
fos.flush();
return true;
} catch (FileNotFoundException e) {
log.error("rar保存文件失败{}", e.getMessage());
return false;
} catch (IOException e) {
log.error("rar保存文件失败{}", e.getMessage());
return false;
} finally {
try {
fos.close();
} catch (IOException e) {
log.error("rar保存文件失败{}", e.getMessage());
}
}
}
评论列表(0条)